Contents
If you are developing in Vue long enough, you will meet a scenario when you need to pass data from child to parent or parent to child. That’s what I’m going to show you in this post. I’ll make it short and to the point so you can continue working on your great project fast.
Passing data from parent to child in Vue
Passing literal value
To pass data from parent to child, you need to use props. Let’s take the following example:
I have a child component called Cow like this:
<template>
<div >
<h3>{{ parentMessage }}</h3>
</div>
</template>
<script>
export default {
name: 'Cow',
props: {
parentMessage: String
}
}
</script>
As you can see, the Cow component has a prop called parentMessage. In the parent component, we can pass data to Cow using this prop.
<template>
<div id="app">
<Cow parentMessage="I am a cow" />
</div>
</template>
Then, you see the message appears as expected:
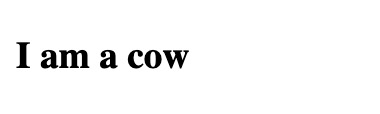
It’s easy, isn’t it?
Updating props
What if you want to change the prop to something else? It’s quite simple, fortunately.
First, let’s create a data property in the parent component called newParentMessage. We are going to bind this property to the Cow’s prop.
In the parent component, it looks like this:
<Cow :parentMessage="newParentMessage" />
You can see that we put the colon at the beginning of the prop. This signifies we are binding the prop to a variable, not literal value as before.
Let’s create a new button in the parent component so when we click on that, the message to Cow component changes.
<button>Change parent message</button>
We need to create a click handler for this button in the parent component. Let’s call it changeParentMethod. Let’s change the message to “I’m just a poor cow”:
changeParentMessage() {
this.newParentMessage = "I'm just a poor cow"
}
We also need to bind the click handler to the button. So, this is the final code of the button:
<button @click="changeParentMessage">Change parent message</button>
Here is what the page look like:
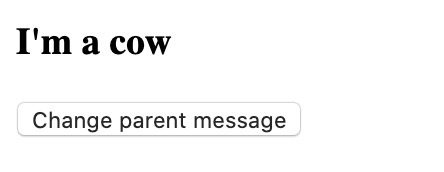
If I click the button now, you’ll see that the message is changed.
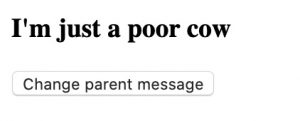
We have successfully pass data from parent to child. Let’s tackle the next challenge: passing data from child component to parent.
When you need to pass data from child to parent in Vue, use $emit. What $emit does it to send a event and in the parent component, you need to have a event handler to catch that event.
Sounds confusing? Let’s see a graph:
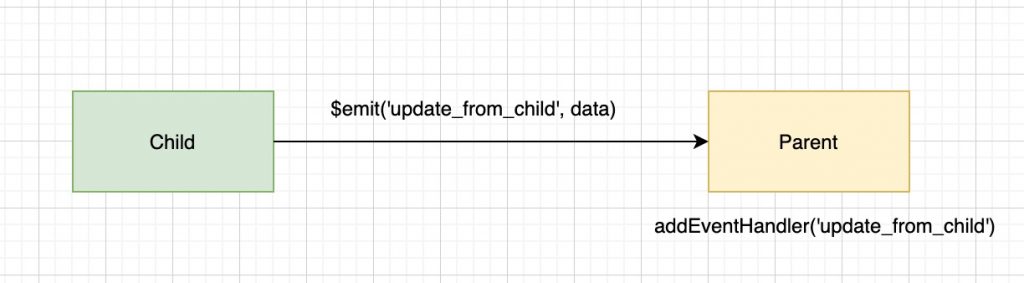
Still confusing? Let’s code!
So currently, the cow message is “I’m just a poor cow”. What if since the start of this post, the cow has been working insanely and got rich? It feels the need to update the status to parent saying: “I’m not poor anymore”.
Let’s help the cow do that. He’s a cool cow.
In the Cow component, let’s create a button so we can send the update. On this button click we will emit an event called update_cow_status along with the data it wants to send.
<button @click="updateFinancialStatus">Update cow status</button>
Next, we need to create the function updateFinancialStatus in Cow component to actually emit the event update_cow_status.
updateFinancialStatus() {
this.$emit('update_cow_status', {
message: "I'm not poor anymore",
netWorth: "$400Bn, with a B!!!"
})
}
As you can see, we emit the event along with a data object. The next task is to get the data in parent.
In the parent component, let’s register a handler for update_cow_status event. This is how you do it:
<Cow @update_cow_status="getCowMessage" :parentMessage="newParentMessage" />
As you can see, you need to put the event name in the Cow component. Let’s create the getCowMessage in parent’s methods. I’ll keep it simple to just log the message from Cow.
getCowMessage(message) {
console.log(message)
}
Now, let’s see our page:
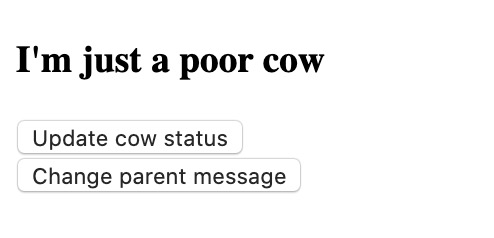
When I click on Update cow status button, I’ll get the following data in the console:

As you can see, we have successfully passed the data from child component to parent with $emit.
Source code
If you need the full source code, you can download it here:
Conclusion
Passing data from child to parent and parent to child is very useful when working on small projects. In larger projects, you might want to use Vuex to store and update data and share such data between components.