Contents
As I develop more and more plugins for WooCommerce, a need for WooCommerce product search emerges. You know, this kind of product search:
If you ever need the full source code of this plugin, please visit this github repo
Let’s learn how to create a field like that so you can use in your plugin.
How to create WooCommerce products search select box
Let’s go through the steps to create a product search input for you plugin:
- Enqueue select2 library
- Create HTML structure
- Write the needed Javascript to enable search
Enqueue select2 library
Select2 is a jQuery plugin that does many amazing things including enable search for traditional select box. It also supports getting list from ajax (which is exactly what we are going to do).
Enqueuing select2 is simple. Let’s create a class for our plugin and put the following code
<?php /** * Plugin Name: BC AJAX Product Search * Plugin URI: https://www.binarycarpenter.com/ * Description: Enable ajax product search * Version: 1.0.0 * Author: BinaryCarpenter.com * Author URI: https://www.binarycarpenter.com * License: GPL2 * Text Domain: bc-ajax-product-search * WC requires at least: 3.0.0 * WC tested up to: 3.6.3 */ class BC_AJAX_Product_Search { public function __construct() { add_action('admin_enqueue_scripts', array($this, 'enqueue_admin')); add_action('admin_menu', array($this, 'add_to_menu')); } public function add_to_menu() { add_menu_page( 'BC Product Search', 'BC Product Search', 'manage_options', 'bc-product-search', array($this,'plugin_ui') ); } public function plugin_ui() { } public function enqueue_admin() { wp_register_style('my-plugin-select2-style', 'https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.10/css/select2.min.css'); wp_enqueue_style('my-plugin-select2-style'); wp_register_script('my-plugin-select2-script', 'https://cdnjs.cloudflare.com/ajax/libs/select2/4.0.10/js/select2.min.js', array('jquery')); wp_register_script('my-plugin-script', plugins_url('static/scripts.js', __FILE__), array('jquery')); wp_enqueue_script('my-plugin-select2-script'); wp_enqueue_script('my-plugin-script'); } } new BC_AJAX_Product_Search();
As you can see, I have enqueue select2 from a CDN. In addition, I also enqueued a Javascript file called scripts.js. This is where we put all our Javascript code.
Finally, I have added a blank function for displaying the UI: plugin_ui. This is the function that output the HTML for the plugin. Let’s do that next.
Create HTML structure
HTML code for this plugin is very simple. We only need a blank select with class. Let’s add that:
public function plugin_ui() { ?> <h1>Select your products</h1> <select data-security="<?php echo wp_create_nonce( 'search-products' ); ?>" multiple style="width: 300px;" class="bc-product-search"></select> <?php }
As you can see, I enabled multiple setting for this select box. You can disable that if you don’t want to.
This is what we have so far:
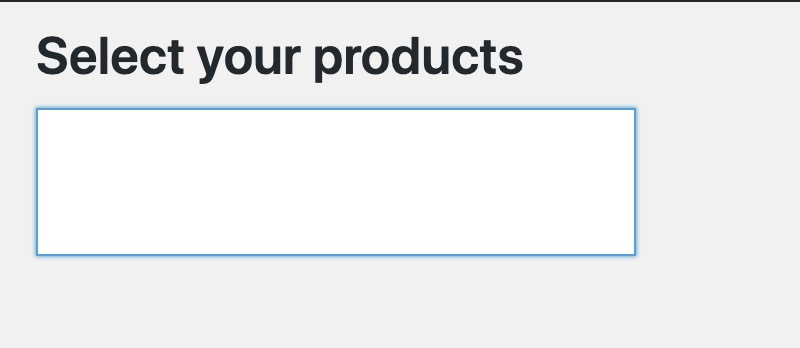
That’s all we need to add for our HTML. Let’s make the product search works with Javascript.Write the needed Javascript to enable search.
Write the needed Javascript to enable search
Let’s put the following code in scripts.js
(function ($) { $(function(){ $('.bc-product-search').select2({ ajax: { url: ajaxurl, data: function (params) { return { term : params.term, action : 'woocommerce_json_search_products_and_variations', security: $(this).attr('data-security'), }; }, processResults: function( data ) { var terms = []; if ( data ) { $.each( data, function( id, text ) { terms.push( { id: id, text: text } ); }); } return { results: terms }; }, cache: true } }); }); })(jQuery)
Save the file and check the plugin. The product search is now working.
How to get data from the select box
If you have used select2 before, getting value from select2 select boxes shouldn’t be alien to you. For those who don’t know, it’s very simple.
Let’s add a button to log the values of the select box:
<button class="show-selected-results">Show select results</button>
$('.show-selected-results').on('click', function(){ console.log($('.bc-product-search').val()); });
Now we have the button:
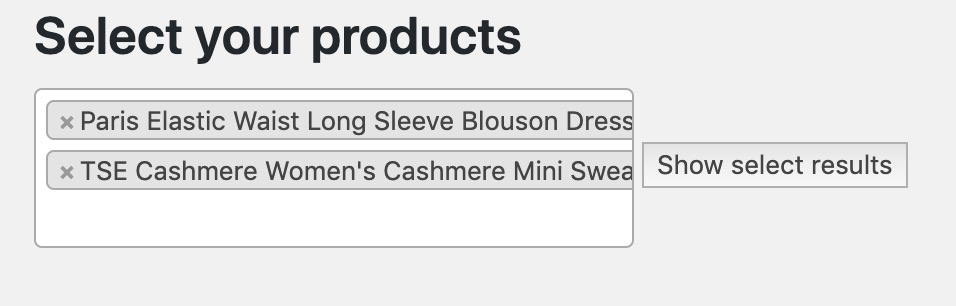
When you click on that button, open the console of your browser, you should see the values:
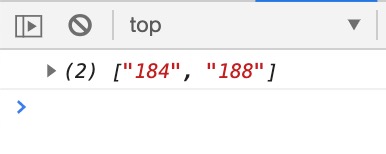
As you can see, they are an array of product ids.
Conclusion
As you can see, it is quite simple to add product search function in your plugin. Hopefully this post is valuable to you. If you have any questions, please let me know.